The forward backward sweep method can be used to solve systems of differential equations, whether they are linear or nonlinear.
A differential equation is an equation that describes how a function changes over time. It involves one or more dependent variables (which are functions of the independent variable, usually time) and their derivatives. A differential equation can be linear or nonlinear depending on whether it is linear in the dependent variables and their derivatives.
The forward-backward sweep method is an algorithm used to find the optimal solution. It’s an iterative approach designed to minimize computational time, and it provides simple results without sacrificing accuracy. Let’s take a look at how this method works.
The Basics of the Forward-Backward Sweep Method
In the forward-backward sweep method, we use two components—the forward sweep and the backward sweep. During the forward sweep, we establish all of our constraints, such as bounds on variables or equality constraints. This information is then used in the backward sweep to solve the problem iteratively until an optimal solution is found. In other words, during the forward sweep, we determine our limits and during the backward sweep we work within those limits to determine our optimal solution.
In the forward-backward sweep method, the “forward sweep” refers to the process of integrating the differential equations from the initial point (the “shooting point”) to the final point, while the “backward sweep” refers to the process of adjusting the initial conditions based on the difference between the predicted and desired values at the final point and then integrating the equations again.
Here is an example of how the forward-backward sweep method can be used to solve a simple linear differential equation:
Consider the differential equation:
dy/dt = -2y + 3
with the initial condition y(0) = 1.
The goal is to find the value of y at time t=1.
- First, we perform a forward sweep by integrating the differential equation from t=0 to t=1 using a numerical integration method such as the Runge-Kutta method. This gives us a predicted value for y at t=1.
- Next, we compare the predicted value for y at t=1 to the desired value (which we have specified in advance). If the error between the two is within a certain tolerance, the solution is considered converged. If the error is too large, we perform a backward sweep to adjust the initial condition y(0) and try again.
- To perform the backward sweep, we compute the sensitivity of the predicted value at t=1 to the initial condition y(0). This can be done using sensitivity analysis or by using an adjoint equation.
- Based on the sensitivity, we adjust the initial condition y(0) and integrate the differential equation again from t=0 to t=1.
- We repeat the forward sweep and backward sweep process until the solution satisfies the boundary condition at t=1 within the desired tolerance.
This process can be generalized to solve systems of linear differential equations, where the initial conditions are adjusted simultaneously and the sensitivity of the predicted values at the final point to the initial conditions is computed using a Jacobian matrix.
Backward Forward Sweep Load Flow Example
The backward-forward sweep method can also be used to solve load flow problems in power networks. In this example, we will use the backward-forward sweep algorithm to solve the AC power flow equations in a simple three-bus network.
The load flow problem requires us to determine the complex voltage magnitudes and angles of each bus in the network to ensure an optimal power flow. This can be done by solving a system of nonlinear equations known as the AC power flow equations.
To solve this system of equations using the backward-forward sweep method, we must first initialize the complex voltages at each bus using an initial guess. Then, we will use the forward sweep to integrate the power flow equations from each bus and compute the resulting voltage magnitudes and angles at all other buses in the network.
Next, we will use a backwards sweep to adjust the voltage magnitude and angle of one or more buses based on differences between these values and their desired values. We repeat this process until convergence is achieved. Once convergence is achieved, we have determined the optimal solution for our load flow problem!
Finally, it’s important to note that while this technique can be used to solve simple linear and nonlinear problems, it can also be applied to more complex problems such as optimal power flow or unit commitment. By combining the forward-backward sweep method with other algorithms or mathematical models, we can solve even more complex problems efficiently.
Backward Forward Sweep Load Flow MATLAB Code
The following MATLAB code shows an example of how the forward backward sweep method can be used to solve a radial distribution network load flow problem, by providing line and load data this MATLAB program can be used to solve IEEE 33, 69 networks
clc,clear all, close all,
format short;
% m=load('loaddata33bus.m');
% l=load('linedata33bus.m');
Pg=zeros(33,1);
Qg=zeros(33,1);
m=load('loaddata33bus.m');
l=load('linedata33bus.m');
br=length(l);
no=length(m);
f=0;
d=0;
MVAb=100;
KVb=12.66;
Zb=(KVb^2)/MVAb;
% Per unit Values
for i=1:br
R(i,1)=(l(i,4))/Zb;
X(i,1)=(l(i,5))/Zb;
end
for i=1:no
P(i,1)=((m(i,2)-Pg(i))/(1000*MVAb));
Pp(i,1)=m(i,2);
Q(i,1)=((m(i,3)-Qg(i))/(1000*MVAb));
Qq(i,1)=m(i,3);
end
Rpp = sum(Pp)
Qpp = sum(Qq)
Apparent_P = sqrt(Rpp^2 + Qpp^2);
PF = Rpp/Apparent_P
R;
X;
P;
Q;
C=zeros(br,no);
for i=1:br
a=l(i,2);
b=l(i,3);
for j=1:no
if a==j
C(i,j)=-1;
end
if b==j
C(i,j)=1;
end
end
end
C;
e=1;
for i=1:no
d=0;
for j=1:br
if C(j,i)==-1
d=1;
end
end
if d==0
endnode(e,1)=i;
e=e+1;
end
end
endnode;
h=length(endnode);
for j=1:h
e=2;
f=endnode(j,1);
% while (f~=1)
for s=1:no
if (f~=1)
k=1;
for i=1:br
if ((C(i,f)==1)&&(k==1))
f=i;
k=2;
end
end
k=1;
for i=1:no
if ((C(f,i)==-1)&&(k==1));
f=i;
g(j,e)=i;
e=e+1;
k=3;
end
end
end
end
end
for i=1:h
g(i,1)=endnode(i,1);
end
g;
w=length(g(1,:));
for i=1:h
j=1;
for k=1:no
for t=1:w
if g(i,t)==k
g(i,t)=g(i,j);
g(i,j)=k;
j=j+1;
end
end
end
end
g;
for k=1:br
e=1;
for i=1:h
for j=1:w-1
if (g(i,j)==k)
if g(i,j+1)~=0
adjb(k,e)=g(i,j+1);
e=e+1;
else
adjb(k,1)=0;
end
end
end
end
end
adjb;
for i=1:br-1
for j=h:-1:1
for k=j:-1:2
if adjb(i,j)==adjb(i,k-1)
adjb(i,j)=0;
end
end
end
end
adjb;
x=length(adjb(:,1));
ab=length(adjb(1,:));
for i=1:x
for j=1:ab
if adjb(i,j)==0 && j~=ab
if adjb(i,j+1)~=0
adjb(i,j)=adjb(i,j+1);
adjb(i,j+1)=0;
end
end
if adjb(i,j)~=0
adjb(i,j)=adjb(i,j)-1;
end
end
end
adjb;
for i=1:x-1
for j=1:ab
adjcb(i,j)=adjb(i+1,j);
end
end
b=length(adjcb);
% voltage current program
for i=1:no
vb(i,1)=1;
end
for s=1:10
for i=1:no
nlc(i,1)=conj(complex(P(i,1),Q(i,1)))/(vb(i,1));
end
nlc;
for i=1:br
Ibr(i,1)=nlc(i+1,1);
end
Ibr;
xy=length(adjcb(1,:));
for i=br-1:-1:1
for k=1:xy
if adjcb(i,k)~=0
u=adjcb(i,k);
%Ibr(i,1)=nlc(i+1,1)+Ibr(k,1);
Ibr(i,1)=Ibr(i,1)+Ibr(u,1);
end
end
end
Ibr;
for i=2:no
g=0;
for a=1:b
if xy>1
if adjcb(a,2)==i-1
u=adjcb(a,1);
vb(i,1)=((vb(u,1))-((Ibr(i-1,1))*(complex((R(i-1,1)),X(i-1,1)))));
g=1;
end
if adjcb(a,3)==i-1
u=adjcb(a,1);
vb(i,1)=((vb(u,1))-((Ibr(i-1,1))*(complex((R(i-1,1)),X(i-1,1)))));
g=1;
end
end
end
if g==0
vb(i,1)=((vb(i-1,1))-((Ibr(i-1,1))*(complex((R(i-1,1)),X(i-1,1)))));
end
end
s=s+1;
end
nlc;
Ibr;
vb;
vbp=[abs(vb) angle(vb)*180/pi];
for i=1:no
vbp(i,1)=abs(vb(i));
vbp(i,2)=angle(vb(i))*(180/pi);
end
for i=1:no
va(i,2:3)=vbp(i,1:2);
end
for i=1:no
va(i,1)=i;
end
va;
Ibrp=[abs(Ibr) angle(Ibr)*180/pi];
PL(1,1)=0;
QL(1,1)=0;
% losses
for f=1:br
Pl(f,1)=(Ibrp(f,1)^2)*R(f,1);
Ql(f,1)=X(f,1)*(Ibrp(f,1)^2);
PL(1,1)=PL(1,1)+Pl(f,1);
QL(1,1)=QL(1,1)+Ql(f,1);
end
Plosskw=(Pl)*100000;
Qlosskw=(Ql)*100000;
PL=(PL)*100000;
QL=(QL)*100000;
voltage = vbp(:,1)
%angle = vbp(:,2)*(pi/180);
% hold on
Ibrp = Ibrp*100000;
Ibrp(:,1)
Plosskw
Qlosskw
PW = sum(Plosskw)
QW = sum(Qlosskw)
%
Plosskw(33,1)=PL;
Qlosskw(33,1)=QL;
Power_Factor = (PW - QW) / PW
VD=sum((1-voltage).^2)*100;
Fit=PL+VD;
%%
% Power Factor = Real Power / Apparent Power
%
% Where:
%
% Real Power = Active Power (kW)
%
% Reactive Power = Reactive Power (kVAr)
%
% Apparent Power = Sqrt(Real Power^2 + Reactive Power^2) (kVA)
%% EXCEL FOR DG
% %EXCEL
% T =table(Sr,Plosskw,Qlosskw,angle,voltage);
% T(:,1:5);
% excel_file = 'NO_DG_IEEE33.xlsx';
% writetable(T,excel_file,'Sheet',1,'Range','H1');
IEEE 33 line and load data is also attached below
% Line Data
% Sr fb tb R X
1 1 2 0.0922 0.0470
2 2 3 0.4930 0.2511
3 3 4 0.3660 0.1864
4 4 5 0.3811 0.1941
4 5 6 0.8190 0.7070
6 6 7 0.1872 0.6188
7 7 8 0.7114 0.2351
8 8 9 1.0300 0.7400
9 9 10 1.0440 0.7400
10 10 11 0.1966 0.0650
11 11 12 0.3744 0.1238
12 12 13 1.4680 1.1550
13 13 14 0.5416 0.7129
14 14 15 0.5910 0.5260
15 15 16 0.7463 0.5450
16 16 17 1.2890 1.7210
17 17 18 0.7320 0.5740
18 2 19 0.2640 0.2565
19 19 20 1.5042 1.3554
20 20 21 0.4095 0.4784
21 21 22 0.7089 0.9373
22 3 23 0.4512 0.3083
23 23 24 0.8980 0.7091
24 24 25 0.8960 0.7011
25 6 26 0.2030 0.1034
26 26 27 0.2842 0.1447
27 27 28 1.0590 0.9337
28 28 29 0.8042 0.7006
29 29 30 0.5075 0.2585
30 30 31 0.9744 0.9630
31 31 32 0.3105 0.3619
32 32 33 0.3410 0.5302
% Load Data
% Sr P Q
1 0 0 0
2 100 60 0
3 90 40 0
4 120 80 0
5 60 30 0
6 60 20 0
7 200 100 0
8 200 100 0
9 60 20 0
10 60 20 0
11 45 30 0
12 60 35 0
13 60 35 0
14 120 80 0
15 60 10 0
16 60 20 0
17 60 20 0
18 90 40 0
19 90 40 0
20 90 40 0
21 90 40 0
22 90 40 0
23 90 50 0
24 420 200 0
25 420 200 0
26 60 25 0
27 60 25 0
28 60 20 0
29 120 70 0
30 200 600 0
31 150 70 0
32 210 100 0
33 60 40 0
The Benefits of Using This Method
There are many benefits associated with using this method to solve nonlinear programming problems. First, it’s computationally efficient because it requires fewer iterations compared to other methods such as sequential quadratic programming (SQP). Additionally, its results are relatively simple and easy to interpret which makes it ideal for complex problems that require multiple variables and constraints. And finally, this algorithm can be used in both unconstrained and constrained optimization problems.
Backward Forward Sweep Method for Radial Distribution System
The backward-forward sweep method is also an effective way to solve radial distribution system problems. Radial distribution systems are networks of interconnected components (such as transmission lines, transformers, and substations) that are used to transfer energy from a specific source to various end users.
When solving IEEE 33 radial distribution system problems using the backward-forward sweep method, the network is divided into two parts: the primary system and the secondary system.
The backward-forward sweep method then works by first solving for the current flowing through each component in the primary system.
Next, voltages at both ends of each transmission line in the primary system are calculated in a forward sweep.
Afterwards, the secondary system is solved by performing a “backward sweep” of current and voltage values from the end of each transmission line in the primary system.
Finally, the current and voltage values at various nodes in the secondary system are calculated by integrating the equations again.
By repeating this process, the desired values at the final point can be calculated and used to optimize system performance.
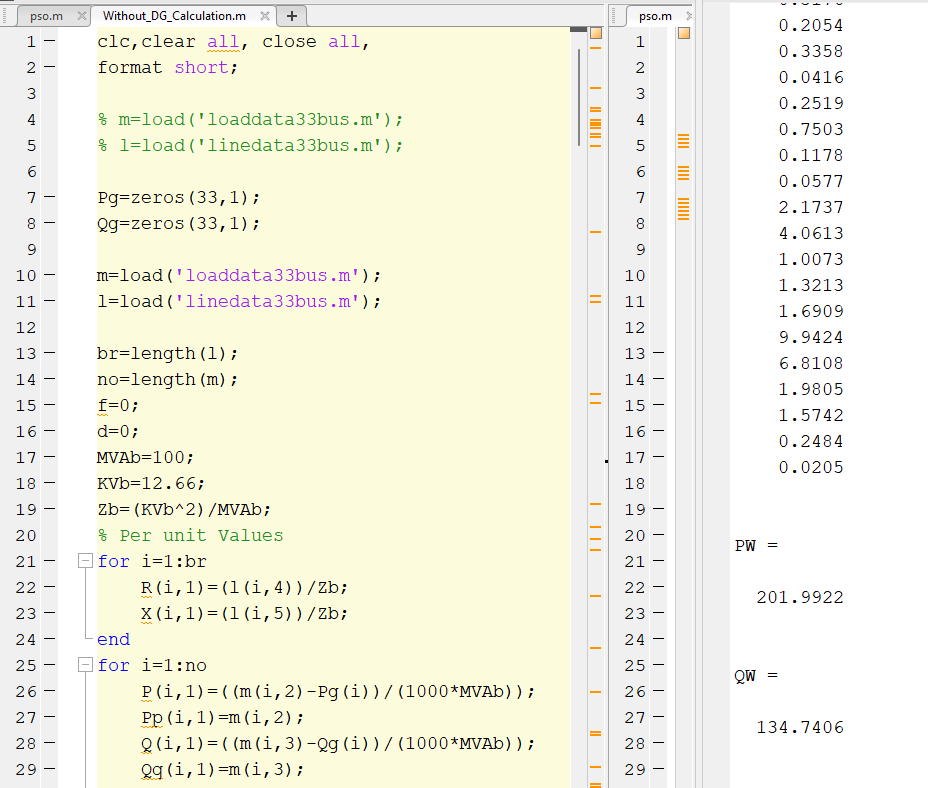
Backward Forward Sweep Method and Gauss Seidel Method
The backward-forward sweep method is similar to the Gauss-Seidel method, which is an iterative method for solving systems of linear equations. Like the backward-forward sweep method, the Gauss-Seidel method involves updating the variables in an orderly fashion (in this case, the variables in the linear equations) and using the updated values to improve the solution at each iteration.
Both the backward-forward sweep method and the Gauss-Seidel method are examples of iterative methods that involve updating variables and using the updated values to improve the solution at each iteration. However, the backward-forward sweep method is specifically used to solve systems of differential equations, while the Gauss-Seidel method is used to solve systems of linear equations.
Downsides of the Forward Backward sweep method
There are a few potential downsides to using the forward-backward sweep method to solve systems of differential equations:
- The method may be computationally intensive, especially for large or complex systems of differential equations. This is because the algorithm involves integrating the equations multiple times and adjusting the initial conditions at each iteration.
- The method may converge slowly or fail to converge altogether if the initial conditions are not chosen carefully. This can be particularly problematic for nonlinear differential equations, which may have multiple solutions or no solutions at all, and may be more sensitive to the initial conditions.
- The method may not be suitable for systems of differential equations that have discontinuities or singularities. These types of equations may require the use of more specialized numerical methods.
- The method assumes that the solution is a smooth function of the initial conditions, which may not always be the case. This can limit the applicability of the method to certain types of problems.
Overall, the forward-backward sweep method can be an effective method for solving systems of differential equations, but it is not always the best choice for every problem. The choice of numerical method should depend on the specific characteristics of the problem being solved.
Conclusion
Overall, if you’re looking for a reliable algorithm that can provide accurate solutions without requiring too much computational power or effort then consider using the forward-backward sweep method. This iterative approach has many advantages including efficiency, simplicity of results, and availability for both constrained and unconstrained optimization problems. So if you’re looking for a reliable optimization algorithm then this might be just what you need!