Hello everybody,
This blog post is about Controller parameters tuning of the DE algorithm and its application to LFC Matlab Code. We have used the following paper’s multi-area system and applied another algorithm to it. In addition to the paper, I have analyzed its robustness by a change in two different parameters of the system, R (governor speed regulation) and B (frequency bias parameter). In addition to the HVDC link, we have considered SMEs. The algorithm I have used is SCA and the paper’s algorithm is DE (differential evolution)
A novel population-based optimization algorithm called Sine Cosine Algorithm (SCA) has been adopted to solve the optimization of automatic load frequency control (LFC) of a realistic power system with multi-source power generation is presented. The sensitivity analysis reveals that the proposed controller is quite robust and optimum controller gains once set for the nominal condition need not be changed for ±25% variations in the system parameters and operating load condition from their nominal values. The dynamic performance of the proposed controller is investigated by the integral of time multiplied absolute error (ITAE) cost function.
Differential Evolution (DE), Artificial Bee Colony (ABC), and Particle Swarm Optimization (PSO) are all heuristic optimization algorithms that can be used to find the global minimum or maximum of a function.
DE is a population-based algorithm that uses a combination of mutation and crossover operations to generate new candidate solutions (called “individuals”) in the search space. ABC is a population-based algorithm that is inspired by the foraging behavior of bees. It uses a set of “bees” to search for the optimal solution. PSO is a population-based algorithm that is inspired by the behavior of flocks of birds. It uses a set of “particles” to search for the optimal solution.
All three algorithms are iterative, meaning that they update the positions of the individuals or particles in the search space over a series of iterations. They all have the ability to find the global minimum or maximum of a function, although they may differ in their efficiency and robustness.
DE, ABC, and PSO have been applied to a wide range of optimization problems, including problems in engineering, finance, and biology. They have been shown to be effective and efficient optimization algorithms in many cases.
Differential Evolution (DE) and Sine Cosine Algorithm
Differential Evolution (DE) and Sine Cosine Algorithm (SCA) are both heuristic optimization algorithms that can be used to find the global minimum or maximum of a function. Both algorithms are iterative, meaning that they update the positions of the individuals or particles in the search space over a series of iterations.
DE is a population-based algorithm that uses a combination of mutation and crossover operations to generate new candidate solutions (called “individuals”) in the search space. SCA is also a population-based algorithm that uses a combination of sine and cosine functions to generate new positions for a set of “particles” in the search space.
Both algorithms have the ability to find the global minimum or maximum of a function, although they may differ in their efficiency and robustness. DE and SCA have been applied to a wide range of optimization problems, including problems in engineering, finance, and biology. They have been shown to be effective and efficient optimization algorithms in many cases.
Differential Evolution Matlab code
Here is a simple example of a Differential Evolution Algorithm Matlab code that implements the Differential Evolution (DE) algorithm:
function [best_solution, best_fitness] = DE(fitness_function, population_size, num_generations, F, CR)
% Differential Evolution (DE) algorithm
%
% Inputs:
% fitness_function - the function to be optimized
% population_size - the number of individuals in the population
% num_generations - the number of generations to evolve the population
% F - the scaling factor
% CR - the crossover probability
%
% Outputs:
% best_solution - the best solution found
% best_fitness - the fitness of the best solution
% Initialize the population with random solutions
pop = rand(population_size, length(fitness_function));
% Evaluate the fitness of the initial solutions
fitness = zeros(population_size, 1);
for i = 1:population_size
fitness(i) = fitness_function(pop(i,:));
end
% Iterate over the generations
for g = 1:num_generations
% Iterate over the individuals in the population
for i = 1:population_size
% Select three other individuals randomly
others = setdiff(1:population_size, i);
r1 = others(randi(length(others)));
r2 = others(randi(length(others)));
r3 = others(randi(length(others)));
% Generate a mutant solution
mutant = pop(r1,:) + F * (pop(r2,:) - pop(r3,:));
% Generate a trial solution by crossover
trial = zeros(1, length(fitness_function));
for j = 1:length(fitness_function)
if rand() <= CR || j == randi(length(fitness_function))
trial(j) = mutant(j);
else
trial(j) = pop(i,j);
end
end
% Evaluate the fitness of the trial solution
trial_fitness = fitness_function(trial);
% If the trial solution is better than the current solution,
% update the current solution
if trial_fitness < fitness(i)
pop(i,:) = trial;
fitness(i) = trial_fitness;
end
end
% Find the best solution in the current population
[best_fitness, best_idx] = min(fitness);
best_solution = pop(best_idx,:);
end
- The function takes as inputs the fitness function to be optimized, the size of the population, the number of generations to evolve the population, and the DE hyperparameters F and CR.
- The population of candidate solutions is initialized with random solutions.
- The fitness of the initial solutions is evaluated using the fitness function.
- The main loop iterates over the generations. In each generation, the function iterates over the individuals in the population.
- For each individual, three other individuals are selected randomly.
- A mutant solution is generated
Sine Cosine Algorithm MATLAB Code
Here is a simple example of a Matlab function that implements the Sine Cosine Algorithm (SCA):
function [best_solution, best_fitness] = SCA(fitness_function, population_size, num_iterations, A, omega)
% Sine Cosine Algorithm (SCA)
%
% Inputs:
% fitness_function - the function to be optimized
% population_size - the number of particles in the population
% num_iterations - the number of iterations to evolve the population
% A - the acceleration coefficient
% omega - the inertia weight
%
% Outputs:
% best_solution - the best solution found
% best_fitness - the fitness of the best solution
% Initialize the population with random solutions
pop = rand(population_size, length(fitness_function));
% Evaluate the fitness of the initial solutions
fitness = zeros(population_size, 1);
for i = 1:population_size
fitness(i) = fitness_function(pop(i,:));
end
% Initialize the velocities of the particles
velocities = zeros(population_size, length(fitness_function));
% Iterate over the iterations
for i = 1:num_iterations
% Update the velocities of the particles
for j = 1:population_size
velocities(j,:) = omega * velocities(j,:) + ...
A * rand() * (best_solution - pop(j,:)) + ...
A * rand() * (best_global_solution - pop(j,:));
end
% Update the positions of the particles
for j = 1:population_size
pop(j,:) = pop(j,:) + velocities(j,:);
end
% Evaluate the fitness of the updated solutions
for j = 1:population_size
fitness(j) = fitness_function(pop(j,:));
end
% Update the best solution and global best solution
[best_fitness, best_idx] = min(fitness);
best_solution = pop(best_idx,:);
[best_global_fitness, best_global_idx] = min(fitness);
best_global_solution = pop(best_global_idx,:);
end
- The function takes as inputs the fitness function to be optimized, the size of the population, the number of iterations to evolve the population, and the SCA hyperparameters A and omega.
- The population of particles is initialized with random solutions.
- The fitness of the initial solutions is evaluated using the fitness function.
- The velocities of the particles are initialized to zero.
- The main loop iterates over the iterations. In each iteration, the function updates the velocities of the particles using the sine and cosine functions.
- The positions of the particles are updated using the velocities.
- The fitness of the updated solutions is evaluated using the fitness function.
- The best solution and global best solution are updated. The best solution is the best solution among the particles in
Automatic generation control in Differential Evolution DE
Differential Evolution (DE) is a heuristic optimization algorithm that can be used to find the global minimum or maximum of a function. It is often used to solve optimization problems that involve finding the optimal values of a set of parameters.
In DE, a population of candidate solutions (called “individuals”) is iteratively updated using a combination of mutation and crossover operations. These operations are performed on the individuals in the population in order to generate new, potentially better solutions. The process of updating the population is known as the “evolution” of the population.
Automatic generation control (AGC) refers to the process of automatically controlling the generation of electric power in a power system in order to match the demand for power with the supply. In DE, AGC could be implemented by using the algorithm to optimize the generation of electric power in a power system. For example, DE could be used to find the optimal values of the generation and dispatch of electric power in order to minimize the cost of generation while meeting the demand for power.
Multi-source power system
Differential Evolution (DE) can be used to optimize the operation of a multi-source power system in a number of ways. For example, DE can be used to:
- Optimize the generation and dispatch of electricity from multiple sources in order to minimize the cost of generation while meeting the demand for power. This can be achieved by using DE to find the optimal values of the generation and dispatch of electricity from each source.
- Optimize the operation of energy storage systems in a multi-source power system. DE can be used to find the optimal values of the charge and discharge rates of the energy storage systems in order to minimize the cost of energy storage while meeting the demand for electricity.
- Optimize the operation of demand-side management systems in a multi-source power system. DE can be used to find the optimal values of the demand-side management strategies (e.g. load shifting, load shedding) in order to minimize the cost of electricity while meeting the demand for power.
Overall, DE can be a useful tool for optimizing the operation of a multi-source power system by finding the optimal values of the various parameters that affect the generation and dispatch of electricity in the system.
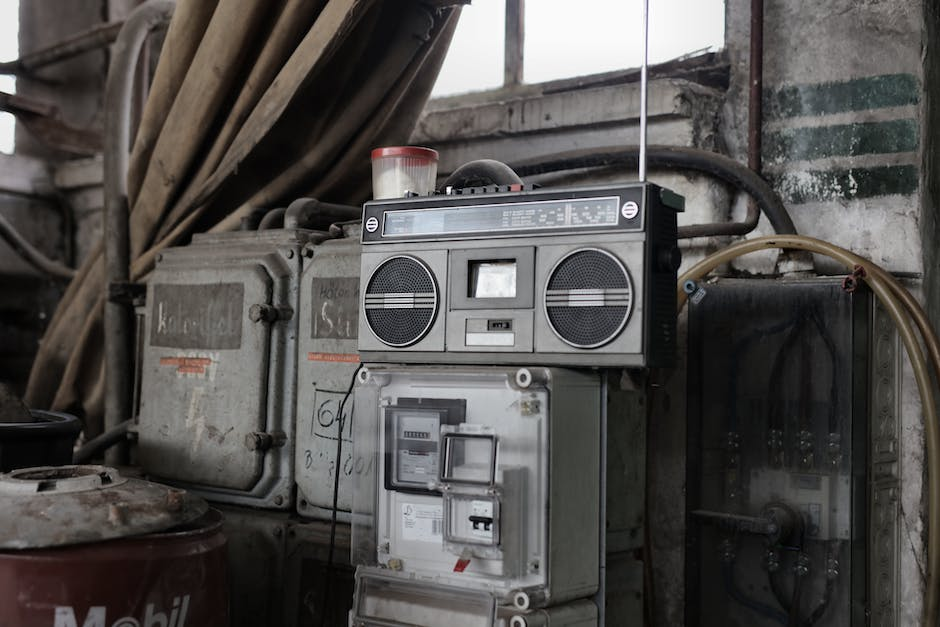
Superconducting Magnetic Energy Storage (SMES)
Superconducting Magnetic Energy Storage (SMES) is a type of energy storage system that uses superconducting materials to store and release electrical energy. In a SMES system, electrical energy is stored in a superconducting coil by creating a magnetic field. The stored energy can then be released back into the electrical grid when needed.
SMES systems have several advantages over other types of energy storage systems. They can store large amounts of energy and release it very quickly, making them well-suited for applications that require fast response times. They also have high energy densities, which means that they can store a lot of energy in a relatively small space. Additionally, SMES systems are very efficient, with low losses of energy during charging and discharging.
One potential application of SMES is in power systems, where it can be used to smooth out fluctuations in the power supply and demand and to provide support to the grid during times of high demand or instability. SMES can also be used in other applications, such as electric vehicles, aircraft, and ships.
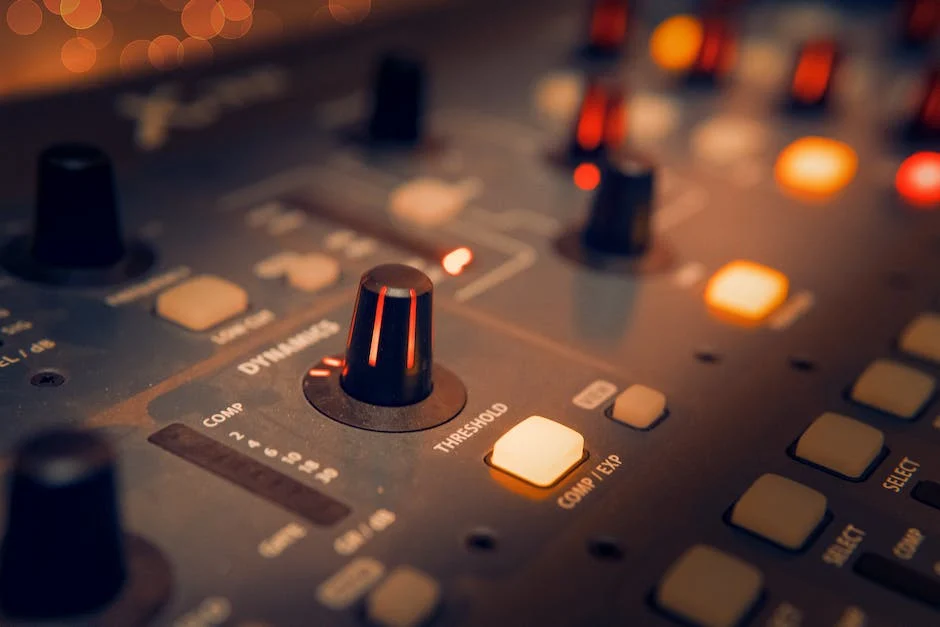
Sine Cosine Algorithm (SCA)
The Sine Cosine Algorithm (SCA) is a heuristic optimization algorithm that is inspired by the sine and cosine functions. It can be used to find the global minimum or maximum of a function.
In SCA, a set of candidate solutions (called “particles”) is iteratively updated using a combination of sine and cosine functions. These functions are used to generate new positions for the particles in the search space. The process of updating the positions of the particles is known as the “movement” of the particles.
SCA has several notable characteristics that distinguish it from other optimization algorithms. It is a simple and easy-to-implement algorithm that does not require the use of any complex mathematical operations. It is also a population-based algorithm, which means that it uses a group of particles to search for the optimal solution. This can make SCA more robust and efficient than some other optimization algorithms that rely on a single particle.
SCA has been applied to a variety of optimization problems, including problems in engineering, finance, and biology. It has been shown to be an effective and efficient optimization algorithm in many cases.
** For the sake of brevity, I just have compared the results. To access the full code, please contact us.
Research Paper Writing Service UK
We as a Simulation Tutor team offer research writing services to students and professionals. They have a team of experienced writers who are knowledgeable in a wide range of academic fields and are skilled at producing high-quality research papers, essays, dissertations, and other written documents. Their services are designed to help students and professionals save time and effort by outsourcing their research and writing tasks to experts. In addition to providing writing services, Simulation Tutor also offers tutoring and consulting services to help clients improve their research skills and understanding of their subject matter. They pride themselves on their commitment to delivering top-notch research writing services that meet the highest standards of quality and professionalism.
Hope it helps. Good luck 😉
hello, I am jamal faraji from university of kashan. I am willing to cooperate with you for writing a paper on your research field. contact with me please .
Hi,It is possible to share your to code me?Best regards
Your email?
alperensari09@gmail.com
Please share the code to me , tanx
jamal.faraji75@gmail.com
hey can you help me out in understanding the implementation of optimisation algorithm in this example.
Your email.
uk.bandaru@gmail.com
Hello,
Greetings
Can you share with me this code? I am sending you my email id:- milanjoshi323@gmail.com
Thank you so much in advance
Milan Joshi
sir, can i get this Matlab Code,plz send it to:
dpkgpt214@gmail.com
sir , can i get this matlab code , plz send it to me
sanil.0202@gmail.com
Respected Sir,
Can i get this code? kindly mail the code to
rinturockers@gmail.com
Can i get this code?
omar.neda88@gmail.com
regards
hello sir can you send me the code please
ayman.id14105885@gmail.com
Can you send code pls?
send me this code at my E-mail address
ebrene2021@gmail.com
thanks